Project Alpha
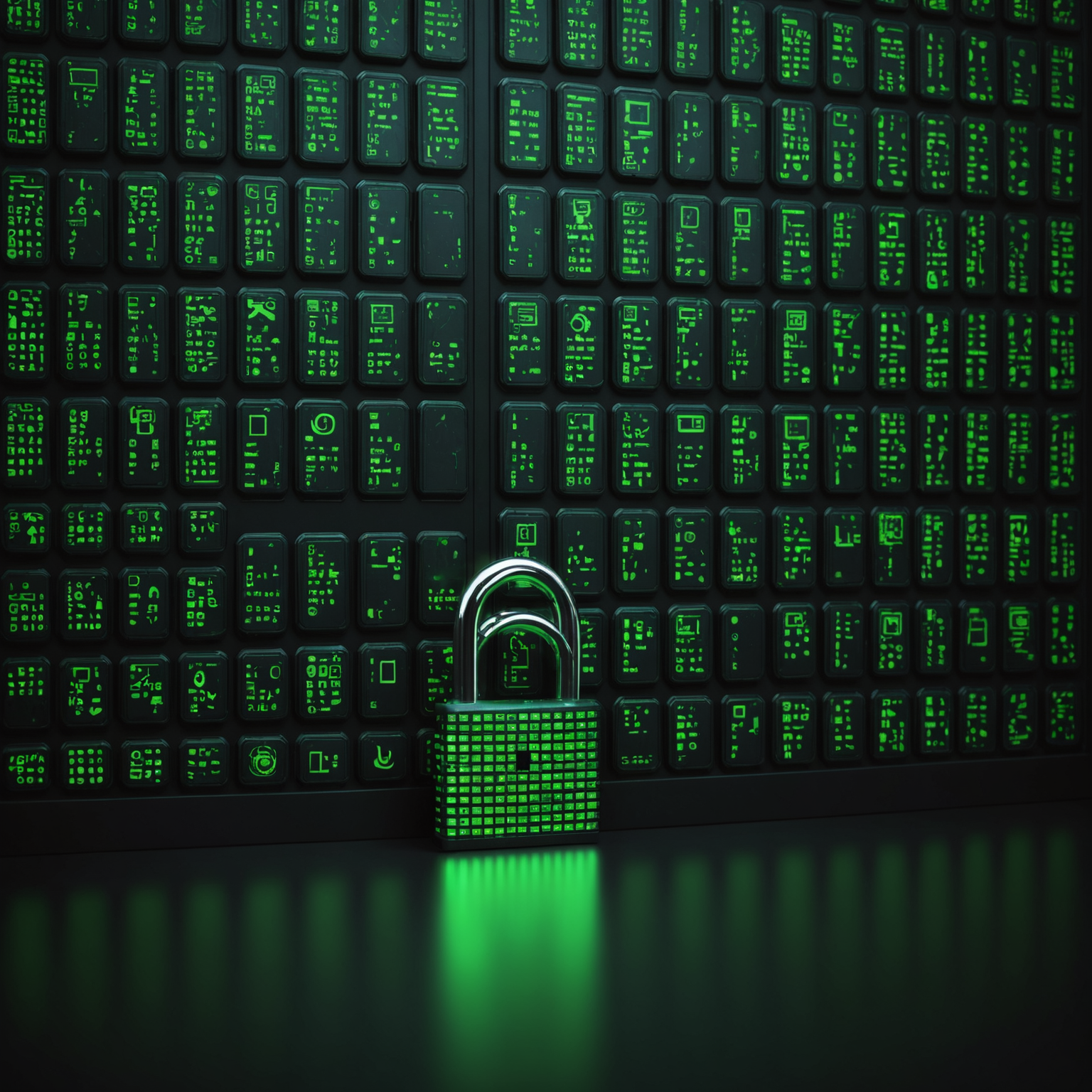
A secure communication platform with end-to-end encryption.
Project Alpha: Secure Communication Platform
Project Alpha is a secure communication platform built with privacy as its core principle. It provides end-to-end encrypted messaging, file sharing, and collaboration tools that ensure your data remains private and secure.
Key Features
End-to-End Encryption
All messages and files are encrypted on the client-side before being transmitted. This means even we can't read your messages:
const encryptMessage = async (message, recipientPublicKey) => {
const encodedMessage = new TextEncoder().encode(message);
// Generate ephemeral key pair for this message
const ephemeralKeyPair = await window.crypto.subtle.generateKey(
{
name: "ECDH",
namedCurve: "P-384",
},
true,
["deriveKey"]
);
// Derive shared secret using recipient's public key
const sharedSecret = await window.crypto.subtle.deriveKey(
{
name: "ECDH",
public: recipientPublicKey,
},
ephemeralKeyPair.privateKey,
{
name: "AES-GCM",
length: 256,
},
false,
["encrypt"]
);
// Encrypt the message with the shared secret
const iv = window.crypto.getRandomValues(new Uint8Array(12));
const encryptedData = await window.crypto.subtle.encrypt(
{
name: "AES-GCM",
iv,
},
sharedSecret,
encodedMessage
);
return {
encryptedData,
iv,
ephemeralPublicKey: ephemeralKeyPair.publicKey,
};
};
Multi-Platform Support
The platform works seamlessly across devices with native applications for:
- Web (React-based SPA)
- iOS (Swift)
- Android (Kotlin)
- Desktop (Electron)
Zero Knowledge Architecture
Our servers are designed with a zero-knowledge approach:
- User authentication via SRP (Secure Remote Password)
- Content is encrypted before it reaches our servers
- Metadata minimization to reduce tracking potential
- Open-source codebase for transparency and auditing
Technical Details
Project Alpha is built with a modern tech stack:
- Frontend: React, TypeScript, and TailwindCSS
- Backend: Node.js with Express
- Database: PostgreSQL with encrypted fields
- Real-time Communication: WebSockets with custom encryption layer
- DevOps: Docker, Kubernetes, and AWS infrastructure
Development Process
The development of Project Alpha followed a security-first approach:
- Initial threat modeling and security architecture design
- Core encryption protocol implementation with peer reviews
- External security audit of cryptographic implementations
- Progressive feature implementation with continuous security testing
- Beta testing with privacy-focused community
- Public release with bug bounty program
Challenges Overcome
Building a secure communication platform presented several challenges:
- Balancing security with usability for non-technical users
- Efficient key management across multiple devices
- Minimizing metadata leakage while maintaining functionality
- Performance optimization for encryption operations on mobile devices
Future Plans
Project Alpha is under active development with several planned features:
- Group encrypted video calls
- Secure file storage with client-side encryption
- Advanced access control for teams
- Hardware security key integration
Conclusion
Project Alpha demonstrates that communication platforms can be both secure and user-friendly. By prioritizing privacy and security from the beginning, we've created a platform that users can trust with their most sensitive communications.